Choosing Between Context API and Redux for Your React Project
An exploration of how to choose the right state management tool—Context API or Redux—based on your project’s needs.
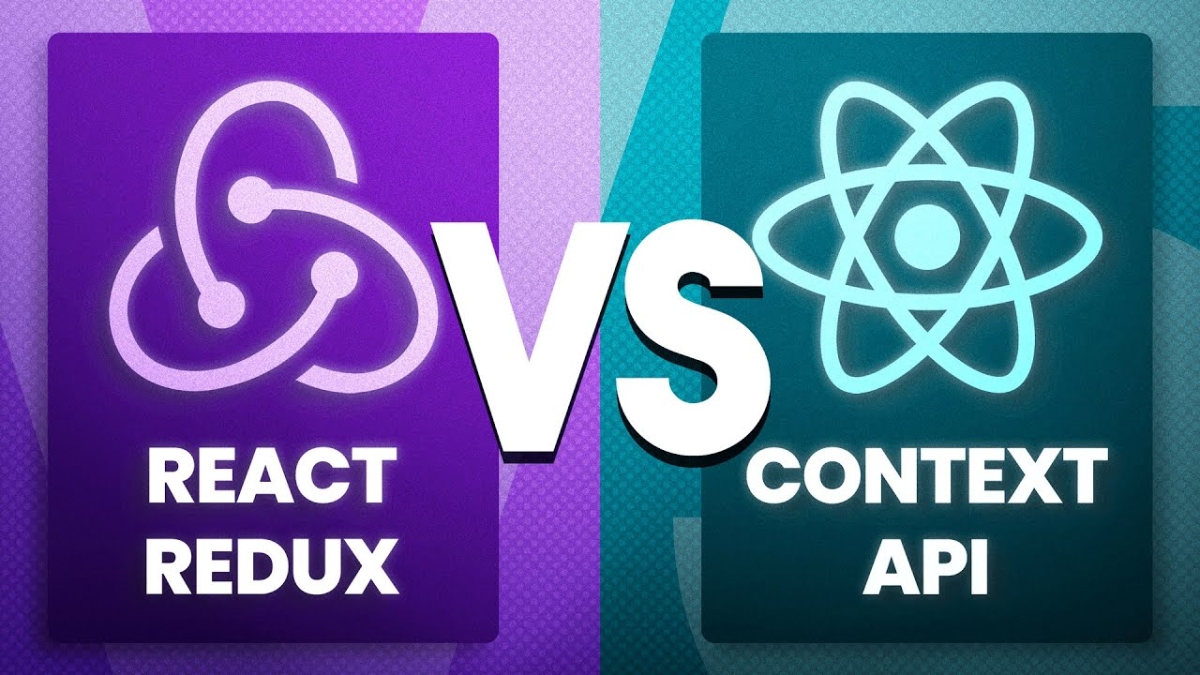
Effective state management is the backbone of any React application. Choosing the right tool—Context API or Redux—can significantly impact both performance and developer experience. Let’s dive into the nuances of these tools, explore when and why you should use them, and discuss how they can shape your project’s journey from functional to exceptional.
What is Context API?
Context API is React’s native solution to the problem of prop drilling. It’s lightweight, straightforward, and designed to handle state sharing across components without unnecessary complexity.
Why Context API?
If your app is small and straightforward, Context API is likely enough. It’s great for managing global states like authentication or theme toggles without adding unnecessary complexity.
Setting Up Context API
-
Create Context: Define a shared space for your data using
createContext
. -
Provide Context: Use the
Provider
component to share the data with your app. -
Consume Context: Retrieve the shared data in any component using
useContext
.
No Installation Needed
Context API comes bundled with React (version 16.3 or later), so you’re ready to roll without additional dependencies.
Code Example: Managing Theme State
import React, { createContext, useContext, useState } from 'react';
const ThemeContext = createContext();
const ThemeProvider = ({ children }) => {
const [theme, setTheme] = useState('light');
const toggleTheme = () => {
setTheme((prevTheme) => (prevTheme === 'light' ? 'dark' : 'light'));
};
return (
<ThemeContext.Provider value={{ theme, toggleTheme }}>
{children}
</ThemeContext.Provider>
);
};
const useTheme = () => useContext(ThemeContext);
const App = () => {
const { theme, toggleTheme } = useTheme();
return (
<div style={{ background: theme === 'light' ? '#fff' : '#333', color: theme === 'light' ? '#000' : '#fff' }}>
<h1>{`Current Theme: ${theme}`}</h1>
<button onClick={toggleTheme}>Toggle Theme</button>
</div>
);
};
export default function Root() {
return (
<ThemeProvider>
<App />
</ThemeProvider>
);
}
What is Redux?
Redux is the heavyweight champion of state management—a centralized, predictable system designed for applications that demand structure and scale. It provides a single source of truth, ensuring that your state management is as clean as a well-maintained library.
Why Redux?
If your app has a lot of interdependent parts and shared state, Redux helps keep everything organized. It simplifies managing complex interactions with a clear, structured approach.
Setting Up Redux
-
Install Redux and React-Redux: Add Redux to your project:
npm install @reduxjs/toolkit react-redux
-
Create a Slice: Use
createSlice
to define state and actions in one place. -
Configure the Store: Combine your slices into a centralized store using
configureStore
. -
Provide the Store: Wrap your app with the
Provider
component to make the store accessible. -
Use Redux Hooks: Fetch state using
useSelector
and update it usinguseDispatch
. This makes working with Redux intuitive and React-friendly.
Code Example: Managing a Shopping Cart
import { configureStore, createSlice } from '@reduxjs/toolkit';
import React from 'react';
import { Provider, useDispatch, useSelector } from 'react-redux';
const cartSlice = createSlice({
name: 'cart',
initialState: [],
reducers: {
addItem: (state, action) => {
state.push(action.payload);
},
removeItem: (state, action) => {
return state.filter((item) => item.id !== action.payload);
},
},
});
const { actions, reducer } = cartSlice;
const { addItem, removeItem } = actions;
const store = configureStore({ reducer: { cart: reducer } });
const ShoppingCart = () => {
const dispatch = useDispatch();
const cart = useSelector((state) => state.cart);
const handleAddItem = () => {
dispatch(addItem({ id: 1, name: 'Item 1', price: 100 }));
};
const handleRemoveItem = (id) => {
dispatch(removeItem(id));
};
return (
<div>
<h1>Shopping Cart</h1>
<button onClick={handleAddItem}>Add Item</button>
<ul>
{cart.map((item) => (
<li key={item.id}>
{item.name} - ${item.price}
<button onClick={() => handleRemoveItem(item.id)}>Remove</button>
</li>
))}
</ul>
</div>
);
};
export default function Root() {
return (
<Provider store={store}>
<ShoppingCart />
</Provider>
);
}
When to Combine Context API and Redux
Sometimes, the best solution is a combination of both. Redux excels in managing complex, large-scale application state with advanced features like middleware and DevTools for debugging.On the other hand, Context API provides an elegant, lightweight solution for localized concerns such as managing UI themes, modal visibility, or authentication state.
By leveraging both tools, you can achieve a balanced approach where Redux powers the core application state while Context API addresses isolated, context-specific requirements.
Decision Matrix
Feature | Context API | Redux |
---|---|---|
Complexity | Low | Moderate to High |
Boilerplate | Minimal | Considerable |
State Debugging | Manual | Advanced (DevTools) |
Middleware Support | Limited | Extensive |
Performance | Context re-renders can affect performance | Optimized with selectors |
Learning Curve | Easy | Steeper |
Scalability | Limited | High |
Conclusion
-
Context API is a simple and lightweight tool best for small projects or straightforward state sharing.
-
Redux is designed for larger applications where managing complex state efficiently is critical.
-
In some scenarios, combining both tools can offer the best of both worlds.
By understanding the strengths and trade-offs of each tool, you’ll be better equipped to make a choice that aligns with your project’s goals and scales gracefully as your application grows.
Why Agile is Not the Silver Bullet for Every Project
The Pitfalls of Blind Agile Adoption and When It Fails
Choosing the Right Database for Your Project
Strategic Database Selection: Aligning Technology with Your Project Vision
The 5 Pillars of Code Quality
Mastering Code Excellence: A Deep Dive into Crafting Scalable, Maintainable, and Secure Software